Projects
Explore my portfolio of bots, APIs, and automation solutions. I specialize in creating WhatsApp bots using Baileys, developing robust APIs, and building custom automation tools with Node.js and JavaScript.
A professional WhatsApp bot service that can be customized for businesses and personal use. Provides automated responses, media handling, and integration with other services.
A versatile bot that works across multiple platforms including Discord, Telegram, and Slack. Uses a unified API to handle messages from different sources.
A solution for businesses to integrate WhatsApp messaging into their customer service workflow using the WhatsApp Business API.
A bot designed to automate testing of web applications. Can simulate user interactions and report issues automatically.
Featured Project: YouTubeDL API
YouTube Downloader API
An API for downloading YouTube videos in MP3 and MP4 formats using Node.js. This project provides a simple way to integrate YouTube video downloading functionality into your applications.
Key Features:
- YouTube search functionality
- MP3 audio download endpoint
- MP4 video download endpoint
- Simple integration with any frontend
- Efficient video processing
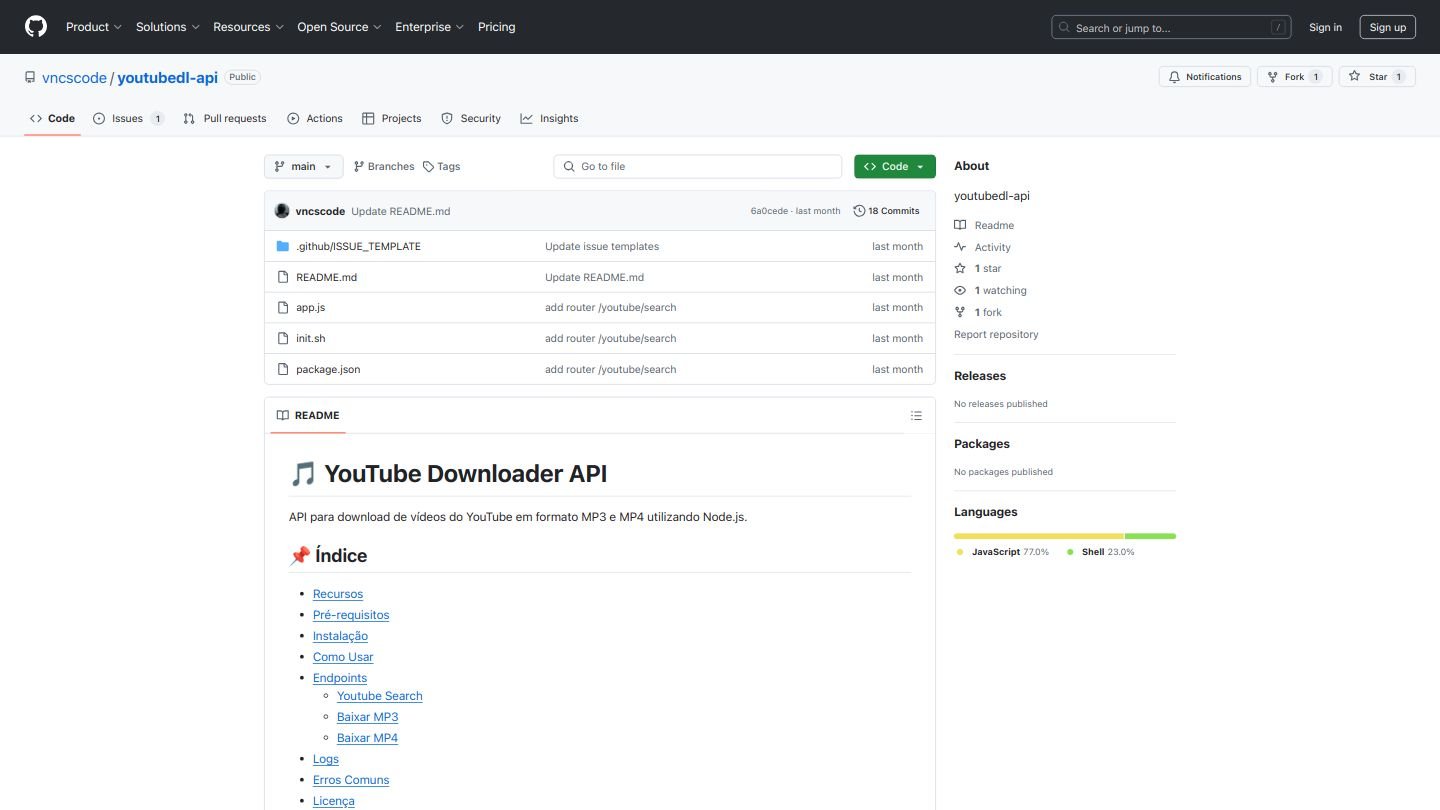
Example Usage
// Search for videos
fetch('/youtube/search?q=your+search+query')
.then(response => response.json())
.then(data => console.log(data));
// Download MP3
fetch('/baixar/mp3?url=https://www.youtube.com/watch?v=videoId')
.then(response => response.blob())
.then(blob => {
// Handle the MP3 file
});
// Download MP4
fetch('/baixar/mp4?url=https://www.youtube.com/watch?v=videoId')
.then(response => response.blob())
.then(blob => {
// Handle the MP4 file
});
WhatsApp Bot Development with Baileys
Key Features
- Multi-device support with the latest WhatsApp protocols
- Message handling with support for text, media, and interactive messages
- Command system with prefix and argument parsing
- Session management and persistence
- Media processing and generation
- Group management capabilities
Contact for Custom Bot
Need a custom WhatsApp bot for your business or personal use? Contact me directly to discuss your requirements.
Contact on WhatsApp: +55 12 98192-1702Technical Expertise
- Deep understanding of the Baileys library and WhatsApp protocols
- Efficient message queue systems to prevent blocking and rate limiting
- Secure authentication and session management
- Scalable architecture for handling multiple instances
- Integration with databases for persistent storage
- Deployment strategies using Docker and cloud services
Join Developer Community
Join my WhatsApp coding group to connect with other developers, share knowledge, and get help with your projects.
Join Coding GroupSample WhatsApp Bot Code
// Basic WhatsApp Bot using Baileys
const { default: makeWASocket, DisconnectReason } = require('@adiwajshing/baileys');
const { Boom } = require('@hapi/boom');
async function connectToWhatsApp() {
const sock = makeWASocket({
printQRInTerminal: true
});
sock.ev.on('connection.update', (update) => {
const { connection, lastDisconnect } = update;
if(connection === 'close') {
const shouldReconnect = (lastDisconnect.error instanceof Boom &&
lastDisconnect.error.output.statusCode !== DisconnectReason.loggedOut);
if(shouldReconnect) {
connectToWhatsApp();
}
}
});
sock.ev.on('messages.upsert', async ({ messages }) => {
const m = messages[0];
if (!m.message) return;
const messageContent = m.message.conversation ||
(m.message.imageMessage && m.message.imageMessage.caption) ||
(m.message.videoMessage && m.message.videoMessage.caption) || '';
if (messageContent.startsWith('!hello')) {
await sock.sendMessage(m.key.remoteJid, { text: 'Hello from my WhatsApp bot!' });
}
});
}